It’s Sunday afternoon, and I just finished writing some important code for Selenium 4.2 (fingers crossed that we can get it released this week). There’s another bug I want to fix, but I’m feeling the need for a break and decide to see what new show I might want to start watching. I visited the site of my favorite TV critic, Alan Sepinwall, to see what he recommends.
In the process of trying to figure out if I want to watch Elisabeth Moss track a time-traveling serial killer, I’m reminded by Rolling Stone that I am reading my final free article. Since I know this, I click to dismiss the reminder, and… I can’t. The down arrow is definitely the thing I need to click, but it isn’t working. I click around until I discover that clicking just under the arrow will collapse the reminder.
This got me thinking about a discussion I’ve been having recently with my friend Nikolay about what kinds of testing companies should be doing and the value of functional DOM to Database tests in general. For instance, Nikolay and I agree that many companies would benefit by converting some of their Selenium tests to API tests. He’s become a big advocate for visual testing recently, though, and has suggested that perhaps a combination of API and visual tests can obviate the need for functional tests. I’m biased, but I’m also not convinced.
What kind of testing would be needed to find the issue I discovered on the Rolling Stone website? First, we should determine if the issue is actually a bug in the first place. I mean, it’s unlikely to affect the company’s bottom line, but it’s annoying. Maybe it’ll annoy my fellow free-loaders enough to make them buy a subscription to the site? Regardless, I was curious about how I might discover the issue with automation; it looks just fine to a visual test, and an API test won’t catch it because nothing gets sent from the DOM.
To see if a functional test would find it, I pulled up my terminal and jumped into an interactive Ruby session:
require 'selenium-webdriver'
driver = Selenium::WebDriver.for :chrome
driver.get 'https://www.rollingstone.com/tv/tv-reviews/shining-girls-review-elisabeth-moss-1339682/'
driver.find_element(class: 'cx-collapse-btn').click
This results in the following error:
element click intercepted: Element <div class="cx-collapse-btn" title="Collapse">...</div>
is not clickable at point (1173, 626).
Other element would receive the click: <div class="cx-content-wrap">...</div>
(Selenium::WebDriver::Error::ElementClickInterceptedError)
So Selenium caught the bug! Selenium attempted to click the the center point of the located element, and couldn’t.
Many Selenium users find “element click intercepted” errors especially frustrating, because the fix isn’t obvious. Common causes and fixes for this error:
- The center of the element is temporarily obscured because the page is still loading—the solution is to wait for the transition to be done and retry.
- The window size is too small for the center of the element to be inside the viewport (this is especially common and non-obvious when testing in headless mode)—increase the window size and/or screen resolution.
- The center of the element is outside the viewport, or hidden under a static header or footer element—scroll the page so the center of the element is inside the viewport and not obscured.
These are all scenarios where it is obvious to a user what needs to be done, but the test code isn’t smart enough to do it automatically. This is what testers get over-conditioned to think in terms of: How is my code not doing what a user would obviously do? This particular situation is the opposite, though; the code is trying to do what a human would do by clicking the center of the element and isn’t able to. The test is failing, but rather than attempting to find a workaround (clicking an adjacent element, clicking with JavaScript, clicking with an offset, etc), this should be flagged as a real problem.
Let’s explore what’s causing this. Firstly, Google’s chromedriver team has provided us with an excellent error message. It tells us which element will intercept the click. Sure enough: The left image here highlights the collapse button that a user would expect to click the center of. The right image highlights the wrapper element that would receive the click instead, and it runs right through the center of the element we want to click.
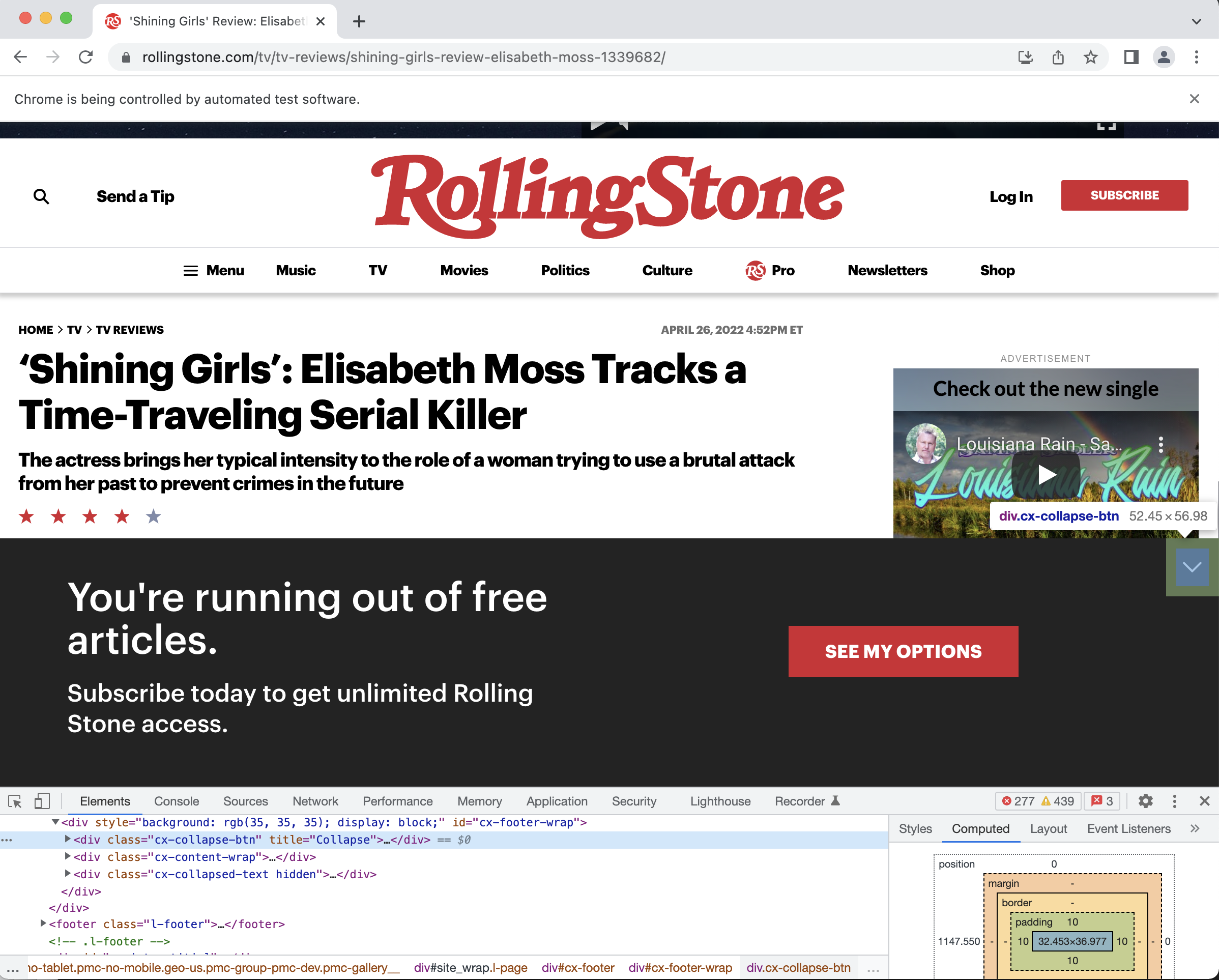
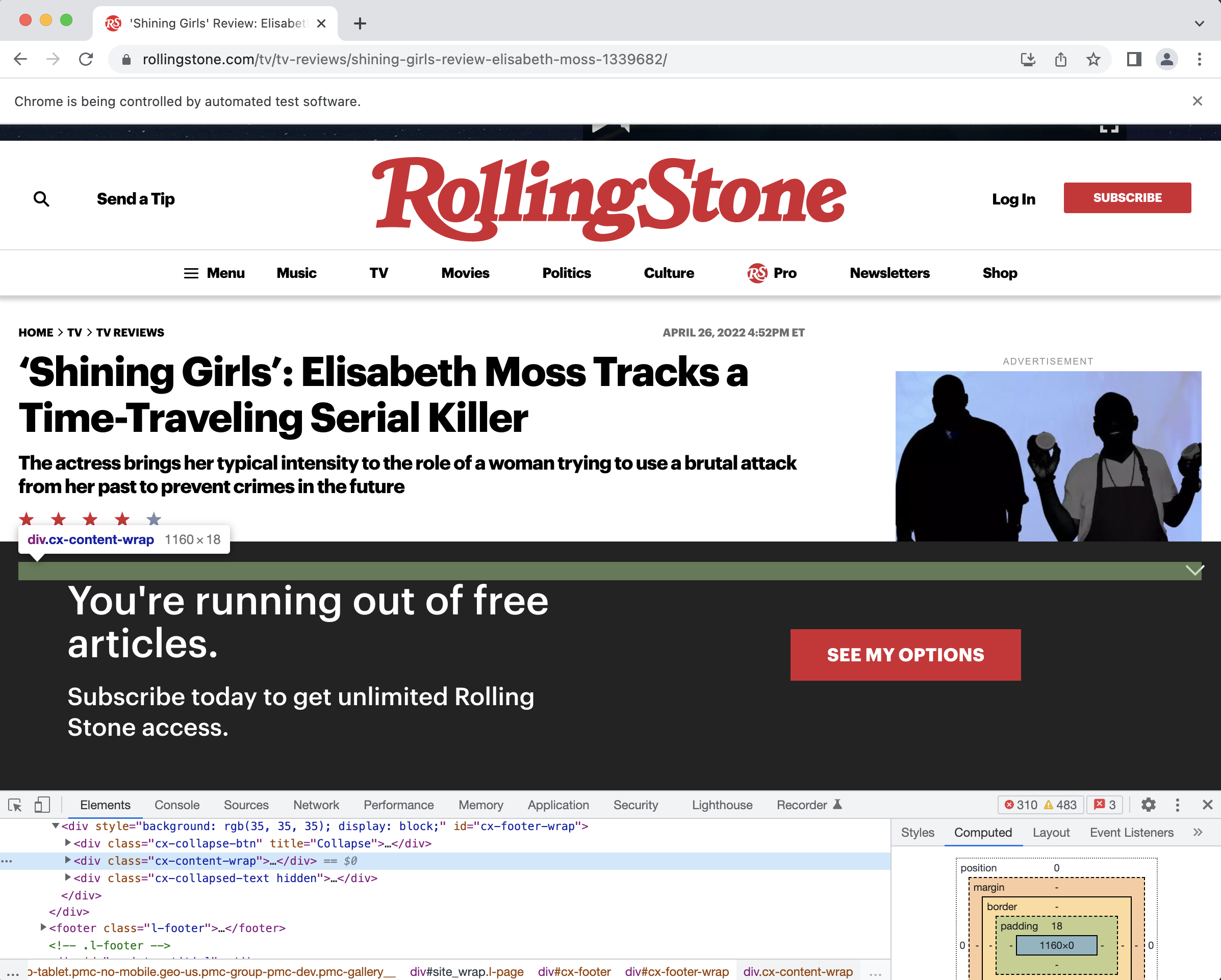
Further, you can see in the lower right hand corner of the second image that this wrapper element has zero height, but has a padding of 18px, which is what is causing this whole mess. This may not be the most consequential bug in the overall scheme of the site, but it’s still worth investigating—maybe this unintended consequence from the padding on a zero-sized dimension is happening somewhere else on the site that is more critical.
The bottom line? I will definitely be watching Shining Girls this week.
or answer one of these questions in the comments or on Twitter: